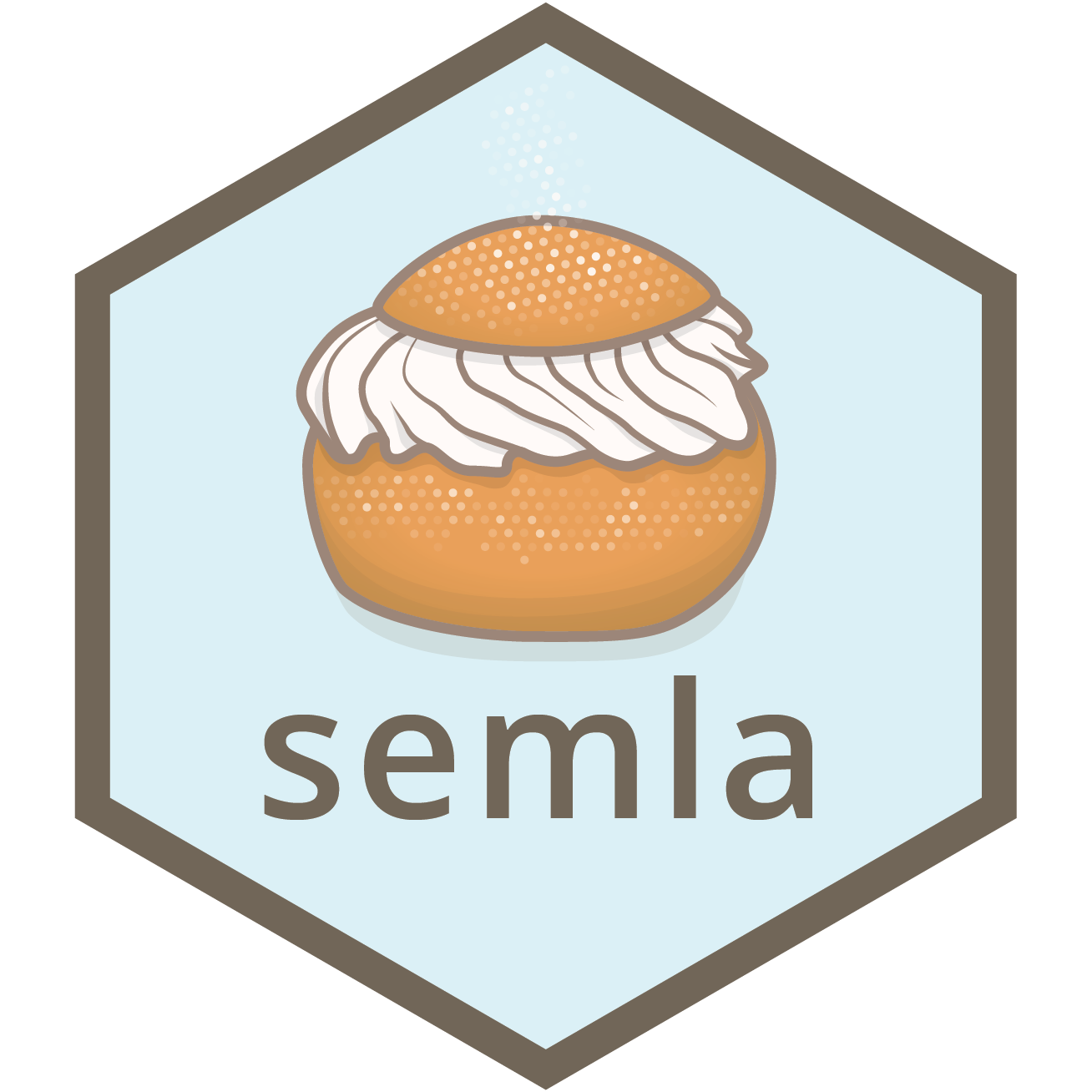
Tile an H&E image
TileImage.Rd
This function takes an image of class magick-image
and create a tile
map. The size of each tile is 256x256 pixels and the number of zoom levels
are determined from the automatically.
Usage
TileImage(
im,
sampleID = 1,
outpath = NULL,
maxZoomLevel = 4,
maxImgWidth = 10000,
nCores = detectCores() - 1,
overwrite = FALSE,
verbose = TRUE
)
Arguments
- im
An image of class
magick-image
- sampleID
The section number to use. This number will be appended to the output files names
- outpath
A string specifying an output directory to save the tiled image in.
- maxZoomLevel
Max zoom level
- maxImgWidth
Safety threshold to make sure that the zoom level doesn't get too deep.
- nCores
Number of cores to use for threading
- overwrite
If the the folder '<outpath>/viewer_data' already exists, it will be overwritten.
- verbose
Print messages
Examples
# \donttest{
library(magick)
library(shiny)
library(leaflet)
#>
#> Attaching package: ‘leaflet’
#> The following object is masked from ‘package:SeuratObject’:
#>
#> JS
#> The following object is masked from ‘package:Seurat’:
#>
#> JS
# Download hires image
he_img <- file.path("https://data.mendeley.com/public-files/datasets/kj3ntnt6vb/files",
"d97fb9ce-eb7d-4c1f-98e0-c17582024a40/file_downloaded")
# Load H&E image with magick
im <- image_read(he_img)
# tile image and return path to tiles
tile_res <- TileImage(im, outpath = tempdir(), nCores = 1, overwrite = TRUE)
#> ℹ Got an image of dimensions 2000x1882 for sample 1
#> → Tiling image into 3 zoom levels
#> ! Replacing directory /private/var/folders/8r/bfm2m_q17znfk0m_8dt330tm0000gp/T/RtmpRVvGCP/viewer_data/tiles1
#> → Creating tiles
#> → Exporting tiles
#> → Exporting meta data
#> ! Replacing file /private/var/folders/8r/bfm2m_q17znfk0m_8dt330tm0000gp/T/RtmpRVvGCP/viewer_data/image_info_1.json
# Create a simple viewer with leaflet
ui <- fluidPage(
leafletOutput("map", height = 512, width = 512),
)
server <- function(input, output, session) {
addResourcePath("mytiles", tile_res$tilepath)
output$map <- renderLeaflet({
leaflet(options = leafletOptions(preferCanvas = TRUE)) %>%
addTiles(urlTemplate = "/mytiles/{z}/{x}_{y}.jpg",
options = tileOptions(continuousWorld = TRUE,
tileSize = "256",
minZoom = 1,
maxZoom = 3))
})
}
if (interactive()) {
shinyApp(ui, server)
}
# }