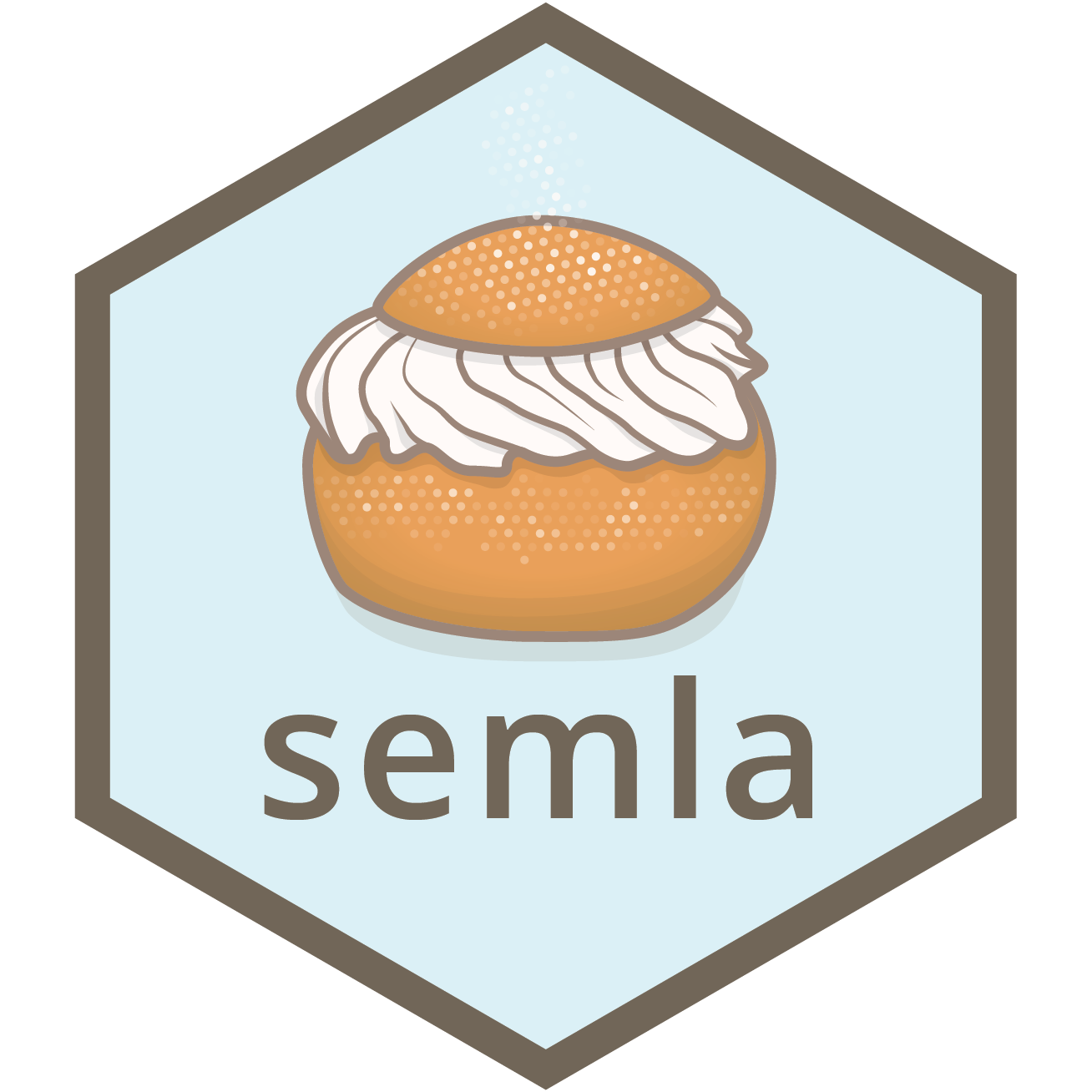
Apply rotations and translations to an image
ImageTransform.Rd
This function takes an magick-image
object as input and applies translations
defined by the angle
and xy_offset
arguments. The output image dimensions
will remain the same as the input image dimensions, meaning that the transformation might
result in cropping the image. If you don't want this behavior, you should use image_rotate
from the magick
R package instead.
Usage
ImageTransform(im, angle = 0, xy_offset = c(0, 0), scalefactor = 1)
Arguments
- im
An image of class
magick-image
- angle
An integer value specifying the rotation angle [-360, 360]
- xy_offset
A numeric vector of length 2 providing the offsets along the x- and y-axis given as pixels. These values should not exceed the image dimensions.
- scalefactor
A numeric value specifying a scaling factor between [0, 3]
Examples
library(magick)
library(semla)
lowresimagefile <- system.file("extdata/mousebrain/spatial",
"tissue_lowres_image.jpg",
package = "semla")
im <- image_read(lowresimagefile)
# rotate image 45 degrees clockwise, move image 100 pixels to the right and 100 pixels down
im_transformed <- ImageTransform(im, angle = 45, xy_offset = c(100, 100))
im_transformed
#> # A tibble: 1 × 7
#> format width height colorspace matte filesize density
#> <chr> <int> <int> <chr> <lgl> <int> <chr>
#> 1 png 565 600 sRGB TRUE 0 72x72
# rotate image 45 degrees counter-clockwise, move image 100 pixels to the left and 20 pixels up
im_transformed <- ImageTransform(im, angle = -45, xy_offset = c(-100, 20))
im_transformed
#> # A tibble: 1 × 7
#> format width height colorspace matte filesize density
#> <chr> <int> <int> <chr> <lgl> <int> <chr>
#> 1 png 565 600 sRGB TRUE 0 72x72
# shrink image to helf width/height
im_transformed <- ImageTransform(im, scalefactor = 0.5)
im_transformed
#> # A tibble: 1 × 7
#> format width height colorspace matte filesize density
#> <chr> <int> <int> <chr> <lgl> <int> <chr>
#> 1 png 565 600 sRGB FALSE 0 72x72