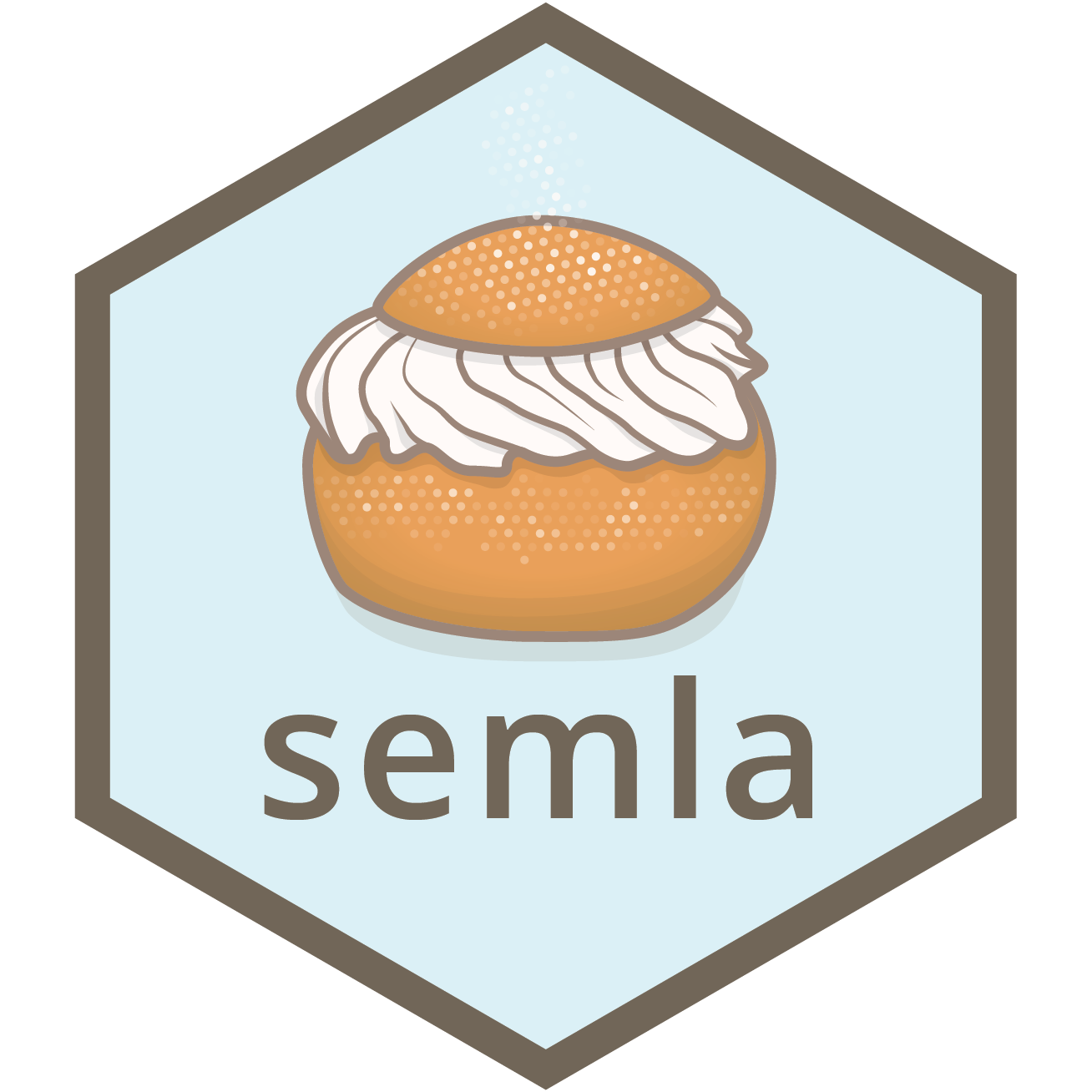
Mirror coordinates
CoordMirror.Rd
Mirror coordinates
Details
The coordinate system for xy_coords
should match the dimensions of the
image. In other words, the coordinates should map spots to the tissue section on H&E image.
A 3x3 transformation matrix is constructed by combining the following matrices:
\(T(-x, -y)\): Translate coordinates to origin, i.e. (0, 0) becomes the new center
1 0 \(-center_{x}\) 0 1 \(-center_{y}\) 0 0 1 \(M_{x}\): Reflect coordinates along x-axis
-1 0 0 0 1 0 0 0 1 \(M_{y}\): Reflect coordinates along y-axis
1 0 0 0 -1 0 0 0 1 \(T(x, y)\): Translate coordinates back to center
1 0 \(center_{x}\) 0 1 \(center_{y}\) 0 0 1
Then, these matrices are combined to form the final transformation matrix:
\(T_{final} = T(x, y)*M_{y}*M_{x}*T(-x, -y)\)
Which can be used to transform our input coordinates:
\(xy_{out} = T_{final}*xy_{in}\)
See also
Other transforms:
CoordAndImageTransform()
,
CoordTransform()
,
ImageTranslate()
,
RigidTransformImages()
,
RunAlignment()
Examples
library(ggplot2)
# Create a data.frame with x, y coordinates
xy <- data.frame(x = 1:20, y = 1:20)
# Reflect coordinates along x axis
xy_mx <- CoordMirror(xy, mirror.x = TRUE) |> setNames(nm = c("x", "y"))
# Reflect along both x, and y axes
xy_mxy <- CoordMirror(xy, mirror.x = TRUE, mirror.y = TRUE) |> setNames(nm = c("x", "y"))
# Combine all coordinates
xy_all <- do.call(rbind, list(cbind(xy, type = "original", ord = 1:20),
cbind(xy_mx, type = "mirror_x", ord = 1:20),
cbind(xy_mxy, type = "mirror_x_and_y", ord = 1:20)))
xy_all$type <- factor(xy_all$type, levels = c("original", "mirror_x", "mirror_x_and_y"))
# Now we can see the effects of mirroring
# Mirror x flips the coordinates along the x axis while mirror_x and mirror_y
# effectively inverts the coordinates, thus changing the order of the points
ggplot(xy_all, aes(x, y)) +
geom_point(color = "steelblue", size = 7, alpha = 0.5) +
geom_text(aes(label = ord)) +
facet_grid(~type) +
geom_vline(xintercept = 10.5, linetype = "dashed", color = "red") +
geom_hline(yintercept = 10.5, linetype = "dashed", color = "green")